Code with Azzan
BLOG
JAvascript• Nodejs • angular •AWS
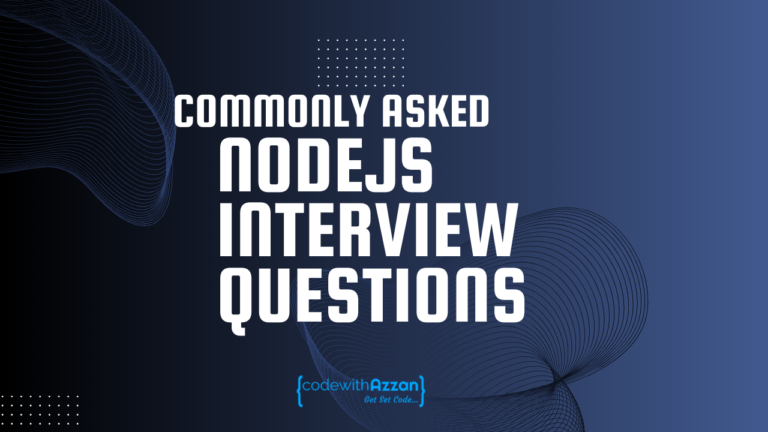
Node.js interview topics
What is Node.js ? Node.js is a powerful open-source JavaScript runtime environment built on Chrome’s V8 JavaScript engine. It allows developers to run JavaScript on the server-side, outside of a web browser. Node.js is asynchronous, event-driven, and non-blocking, which makes it…
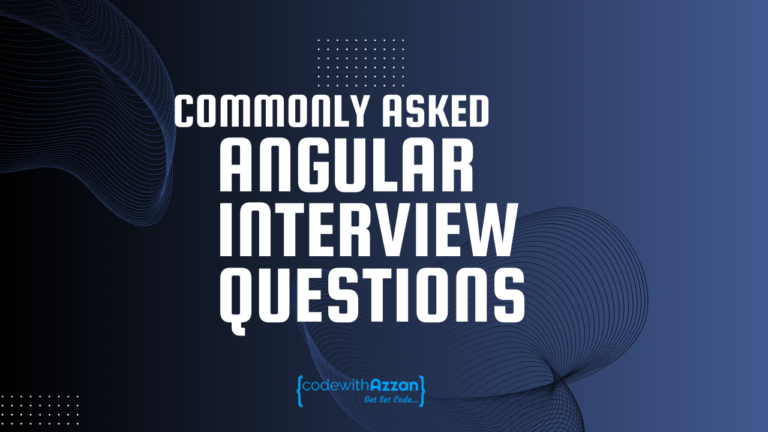
Angular interview topics
What is Angular? Angular is a development platform, built on TypeScript, for building scalable web applications with components, libraries, and tools. It was introduced to create Single Page Applications (SPAs) and bring structure and consistency to web applications, providing excellent scalability…
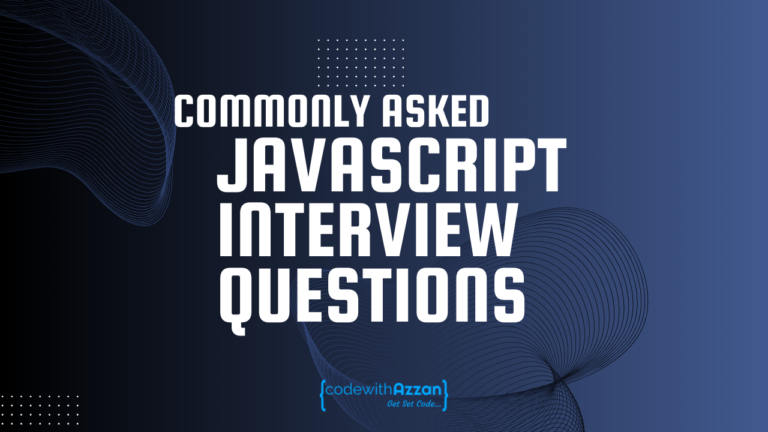
Commonly asked JavaScript Interview Questions (with answers)
What are new ES6 features ? Here’s a summary of key features introduced in ES6 (ECMAScript 2015) that significantly enhanced JavaScript development: 1. Classes: Syntactic sugar for creating object blueprints with inheritance and encapsulation. 2. Arrow Functions: Concise syntax for…
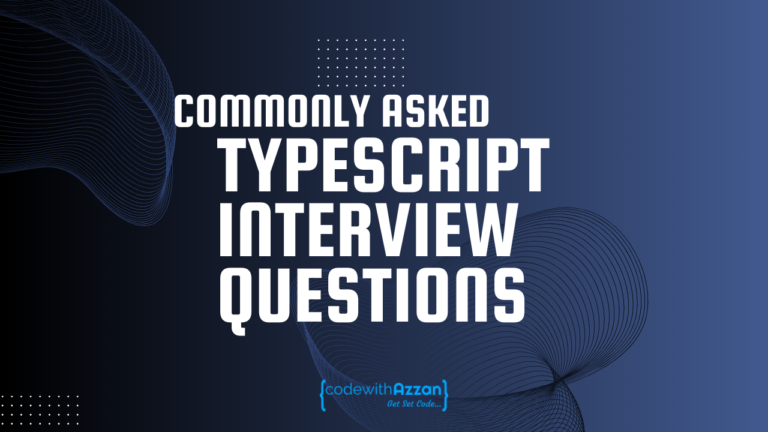
Commonly asked TypeScript Interview Questions (with answers)
What is TypeScript and how does it differ from JavaScript ? Here’s a breakdown of TypeScript and its key differences from JavaScript: TypeScript: Key Differences from JavaScript: What are enums ? In TypeScript, enums are a way to define a set of named…

Using AWS EC2 to Deploy NodeJS and MongoDB Application
Any application that we develop cannot just sit on our local machines and has to be deployed somewhere for others to access it, and cloud seems like an ideal and cost effective choice to do that. So, in this article…

Using WebSockets in NodeJS and Building a Chat App
In this article, we will learn about websockets, what is it, how do we use it and much more. We will also create a NodeJS application demonstrating how to work with websockets and also build another NodeJS application around one…

Using TypeScript to build NodeJS and Express Application
In this article we will learn how can we build a NodeJS and express application using TypeScript, which is being treated as a JavaScript replacement and many NodeJS applications have already started shifting their code from JavaScript to TypeScript. So…

Using Docker to Containerize NodeJS and MongoDB Application
In this article we will learn how to run NodeJS applications on docker. We will take two examples of node applications, one a simple node app returning just ‘Hello from docker!!’ message as response, and another a full fledged node…

Using Swagger to Autogenerate OpenAPI Specifications for REST APIs
In this article we will learn how we can auto generate OpenAPI Specifications using Swagger for RESTful APIs which helps us to describe, consume and visualize the APIs. With the help of swagger we will learn to autogenerate API documentation…

User Authentication in NodeJS using Passport.js (local strategy)
In this article we will learn how to implement user authentication in NodeJS using passport.js which is a authentication middleware and is extremely flexible and modular. In this tutorial we will create a simple NodeJS backend using express with just…

Implementing CRUD in NodeJS with Express and MongoDB: 5 Easy Steps
In this article we will learn how to implement CRUD in NodeJS using MongoDB as database. We will also learn how to build REST APIs for each CRUD operation and to use rest client in visual studio code to test…

Using JWT Refresh Token in NodeJS and Angular Authentication
In this article we will learn how to generate and make use of JWT refresh token to request for new access token without having the need to login every time the access token expires. We will also learn how to…

User Registration, Login and JWT Authentication in Angular
In this article we will learn how to implement user registration, login and JWT authentication in Angular. We will create a simple angular application which will allow user to register, login and then allow user to access application’s home page…

Implementing JWT Authentication in NodeJS
In this article we will learn about implementing JWT authentication in NodeJs using simple and easy to understand code and clean code practices. Every application nowadays has some sort of authorization and authentication as most of the applications require registration…